mirror of
https://github.moeyy.xyz/https://github.com/trekhleb/javascript-algorithms.git
synced 2024-12-26 23:21:18 +08:00
Merge branches 'issue-102-rabin-karp-fix' and 'master' of https://github.com/trekhleb/javascript-algorithms into issue-102-rabin-karp-fix
This commit is contained in:
commit
650e3099e5
@ -48,7 +48,7 @@ _Read this in other languages:_
|
|||||||
|
|
||||||
## 알고리즘
|
## 알고리즘
|
||||||
|
|
||||||
알고리즘은 어떤 종료의 문제를 풀 수 있는 정확한 방법이며,
|
알고리즘은 어떤 종류의 문제를 풀 수 있는 정확한 방법이며,
|
||||||
일련의 작업을 정확하게 정의해 놓은 규칙들입니다.
|
일련의 작업을 정확하게 정의해 놓은 규칙들입니다.
|
||||||
|
|
||||||
`B` - 입문자, `A` - 숙련자
|
`B` - 입문자, `A` - 숙련자
|
||||||
|
@ -10,7 +10,7 @@ export default function kruskal(graph) {
|
|||||||
// It should fire error if graph is directed since the algorithm works only
|
// It should fire error if graph is directed since the algorithm works only
|
||||||
// for undirected graphs.
|
// for undirected graphs.
|
||||||
if (graph.isDirected) {
|
if (graph.isDirected) {
|
||||||
throw new Error('Prim\'s algorithms works only for undirected graphs');
|
throw new Error('Kruskal\'s algorithms works only for undirected graphs');
|
||||||
}
|
}
|
||||||
|
|
||||||
// Init new graph that will contain minimum spanning tree of original graph.
|
// Init new graph that will contain minimum spanning tree of original graph.
|
||||||
|
@ -15,20 +15,20 @@ function combinationSumRecursive(
|
|||||||
) {
|
) {
|
||||||
if (remainingSum < 0) {
|
if (remainingSum < 0) {
|
||||||
// By adding another candidate we've gone below zero.
|
// By adding another candidate we've gone below zero.
|
||||||
// This would mean that last candidate was not acceptable.
|
// This would mean that the last candidate was not acceptable.
|
||||||
return finalCombinations;
|
return finalCombinations;
|
||||||
}
|
}
|
||||||
|
|
||||||
if (remainingSum === 0) {
|
if (remainingSum === 0) {
|
||||||
// In case if after adding the previous candidate out remaining sum
|
// If after adding the previous candidate our remaining sum
|
||||||
// became zero we need to same current combination since it is one
|
// became zero - we need to save the current combination since it is one
|
||||||
// of the answer we're looking for.
|
// of the answers we're looking for.
|
||||||
finalCombinations.push(currentCombination.slice());
|
finalCombinations.push(currentCombination.slice());
|
||||||
|
|
||||||
return finalCombinations;
|
return finalCombinations;
|
||||||
}
|
}
|
||||||
|
|
||||||
// In case if we haven't reached zero yet let's continue to add all
|
// If we haven't reached zero yet let's continue to add all
|
||||||
// possible candidates that are left.
|
// possible candidates that are left.
|
||||||
for (let candidateIndex = startFrom; candidateIndex < candidates.length; candidateIndex += 1) {
|
for (let candidateIndex = startFrom; candidateIndex < candidates.length; candidateIndex += 1) {
|
||||||
const currentCandidate = candidates[candidateIndex];
|
const currentCandidate = candidates[candidateIndex];
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Bloom Filter
|
# Bloom Filter
|
||||||
|
|
||||||
A bloom filter is a space-efficient probabilistic
|
A **bloom filter** is a space-efficient probabilistic
|
||||||
data structure designed to test whether an element
|
data structure designed to test whether an element
|
||||||
is present in a set. It is designed to be blazingly
|
is present in a set. It is designed to be blazingly
|
||||||
fast and use minimal memory at the cost of potential
|
fast and use minimal memory at the cost of potential
|
||||||
|
@ -213,6 +213,16 @@ export default class DoublyLinkedList {
|
|||||||
return nodes;
|
return nodes;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* @param {*[]} values - Array of values that need to be converted to linked list.
|
||||||
|
* @return {DoublyLinkedList}
|
||||||
|
*/
|
||||||
|
fromArray(values) {
|
||||||
|
values.forEach(value => this.append(value));
|
||||||
|
|
||||||
|
return this;
|
||||||
|
}
|
||||||
|
|
||||||
/**
|
/**
|
||||||
* @param {function} [callback]
|
* @param {function} [callback]
|
||||||
* @return {string}
|
* @return {string}
|
||||||
|
@ -36,6 +36,13 @@ describe('DoublyLinkedList', () => {
|
|||||||
expect(linkedList.toString()).toBe('3,2,1');
|
expect(linkedList.toString()).toBe('3,2,1');
|
||||||
});
|
});
|
||||||
|
|
||||||
|
it('should create linked list from array', () => {
|
||||||
|
const linkedList = new DoublyLinkedList();
|
||||||
|
linkedList.fromArray([1, 1, 2, 3, 3, 3, 4, 5]);
|
||||||
|
|
||||||
|
expect(linkedList.toString()).toBe('1,1,2,3,3,3,4,5');
|
||||||
|
});
|
||||||
|
|
||||||
it('should delete node by value from linked list', () => {
|
it('should delete node by value from linked list', () => {
|
||||||
const linkedList = new DoublyLinkedList();
|
const linkedList = new DoublyLinkedList();
|
||||||
|
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Graph
|
# Graph
|
||||||
|
|
||||||
In computer science, a graph is an abstract data type
|
In computer science, a **graph** is an abstract data type
|
||||||
that is meant to implement the undirected graph and
|
that is meant to implement the undirected graph and
|
||||||
directed graph concepts from mathematics, specifically
|
directed graph concepts from mathematics, specifically
|
||||||
the field of graph theory
|
the field of graph theory
|
||||||
|
@ -1,9 +1,9 @@
|
|||||||
# Hash Table
|
# Hash Table
|
||||||
|
|
||||||
In computing, a hash table (hash map) is a data
|
In computing, a **hash table** (hash map) is a data
|
||||||
structure which implements an associative array
|
structure which implements an *associative array*
|
||||||
abstract data type, a structure that can map keys
|
abstract data type, a structure that can *map keys
|
||||||
to values. A hash table uses a hash function to
|
to values*. A hash table uses a *hash function* to
|
||||||
compute an index into an array of buckets or slots,
|
compute an index into an array of buckets or slots,
|
||||||
from which the desired value can be found
|
from which the desired value can be found
|
||||||
|
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Heap (data-structure)
|
# Heap (data-structure)
|
||||||
|
|
||||||
In computer science, a heap is a specialized tree-based
|
In computer science, a **heap** is a specialized tree-based
|
||||||
data structure that satisfies the heap property described
|
data structure that satisfies the heap property described
|
||||||
below.
|
below.
|
||||||
|
|
||||||
|
@ -65,7 +65,8 @@ export default class LinkedList {
|
|||||||
|
|
||||||
let deletedNode = null;
|
let deletedNode = null;
|
||||||
|
|
||||||
// If the head must be deleted then make 2nd node to be a head.
|
// If the head must be deleted then make next node that is differ
|
||||||
|
// from the head to be a new head.
|
||||||
while (this.head && this.compare.equal(this.head.value, value)) {
|
while (this.head && this.compare.equal(this.head.value, value)) {
|
||||||
deletedNode = this.head;
|
deletedNode = this.head;
|
||||||
this.head = this.head.next;
|
this.head = this.head.next;
|
||||||
@ -127,9 +128,10 @@ export default class LinkedList {
|
|||||||
* @return {LinkedListNode}
|
* @return {LinkedListNode}
|
||||||
*/
|
*/
|
||||||
deleteTail() {
|
deleteTail() {
|
||||||
|
const deletedTail = this.tail;
|
||||||
|
|
||||||
if (this.head === this.tail) {
|
if (this.head === this.tail) {
|
||||||
// There is only one node in linked list.
|
// There is only one node in linked list.
|
||||||
const deletedTail = this.tail;
|
|
||||||
this.head = null;
|
this.head = null;
|
||||||
this.tail = null;
|
this.tail = null;
|
||||||
|
|
||||||
@ -137,7 +139,6 @@ export default class LinkedList {
|
|||||||
}
|
}
|
||||||
|
|
||||||
// If there are many nodes in linked list...
|
// If there are many nodes in linked list...
|
||||||
const deletedTail = this.tail;
|
|
||||||
|
|
||||||
// Rewind to the last node and delete "next" link for the node before the last one.
|
// Rewind to the last node and delete "next" link for the node before the last one.
|
||||||
let currentNode = this.head;
|
let currentNode = this.head;
|
||||||
@ -174,6 +175,16 @@ export default class LinkedList {
|
|||||||
return deletedHead;
|
return deletedHead;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* @param {*[]} values - Array of values that need to be converted to linked list.
|
||||||
|
* @return {LinkedList}
|
||||||
|
*/
|
||||||
|
fromArray(values) {
|
||||||
|
values.forEach(value => this.append(value));
|
||||||
|
|
||||||
|
return this;
|
||||||
|
}
|
||||||
|
|
||||||
/**
|
/**
|
||||||
* @return {LinkedListNode[]}
|
* @return {LinkedListNode[]}
|
||||||
*/
|
*/
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Linked List
|
# Linked List
|
||||||
|
|
||||||
In computer science, a linked list is a linear collection
|
In computer science, a **linked list** is a linear collection
|
||||||
of data elements, in which linear order is not given by
|
of data elements, in which linear order is not given by
|
||||||
their physical placement in memory. Instead, each
|
their physical placement in memory. Instead, each
|
||||||
element points to the next. It is a data structure
|
element points to the next. It is a data structure
|
||||||
|
@ -184,6 +184,13 @@ describe('LinkedList', () => {
|
|||||||
expect(linkedList.find({ callback: value => value.key === 'test5' })).toBeNull();
|
expect(linkedList.find({ callback: value => value.key === 'test5' })).toBeNull();
|
||||||
});
|
});
|
||||||
|
|
||||||
|
it('should create linked list from array', () => {
|
||||||
|
const linkedList = new LinkedList();
|
||||||
|
linkedList.fromArray([1, 1, 2, 3, 3, 3, 4, 5]);
|
||||||
|
|
||||||
|
expect(linkedList.toString()).toBe('1,1,2,3,3,3,4,5');
|
||||||
|
});
|
||||||
|
|
||||||
it('should find node by means of custom compare function', () => {
|
it('should find node by means of custom compare function', () => {
|
||||||
const comparatorFunction = (a, b) => {
|
const comparatorFunction = (a, b) => {
|
||||||
if (a.customValue === b.customValue) {
|
if (a.customValue === b.customValue) {
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Priority Queue
|
# Priority Queue
|
||||||
|
|
||||||
In computer science, a priority queue is an abstract data type
|
In computer science, a **priority queue** is an abstract data type
|
||||||
which is like a regular queue or stack data structure, but where
|
which is like a regular queue or stack data structure, but where
|
||||||
additionally each element has a "priority" associated with it.
|
additionally each element has a "priority" associated with it.
|
||||||
In a priority queue, an element with high priority is served before
|
In a priority queue, an element with high priority is served before
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Queue
|
# Queue
|
||||||
|
|
||||||
In computer science, a queue is a particular kind of abstract data
|
In computer science, a **queue** is a particular kind of abstract data
|
||||||
type or collection in which the entities in the collection are
|
type or collection in which the entities in the collection are
|
||||||
kept in order and the principle (or only) operations on the
|
kept in order and the principle (or only) operations on the
|
||||||
collection are the addition of entities to the rear terminal
|
collection are the addition of entities to the rear terminal
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Stack
|
# Stack
|
||||||
|
|
||||||
In computer science, a stack is an abstract data type that serves
|
In computer science, a **stack** is an abstract data type that serves
|
||||||
as a collection of elements, with two principal operations:
|
as a collection of elements, with two principal operations:
|
||||||
|
|
||||||
* **push**, which adds an element to the collection, and
|
* **push**, which adds an element to the collection, and
|
||||||
|
@ -6,7 +6,7 @@
|
|||||||
* [Segment Tree](segment-tree) - with min/max/sum range queries examples
|
* [Segment Tree](segment-tree) - with min/max/sum range queries examples
|
||||||
* [Fenwick Tree](fenwick-tree) (Binary Indexed Tree)
|
* [Fenwick Tree](fenwick-tree) (Binary Indexed Tree)
|
||||||
|
|
||||||
In computer science, a tree is a widely used abstract data
|
In computer science, a **tree** is a widely used abstract data
|
||||||
type (ADT) — or data structure implementing this ADT—that
|
type (ADT) — or data structure implementing this ADT—that
|
||||||
simulates a hierarchical tree structure, with a root value
|
simulates a hierarchical tree structure, with a root value
|
||||||
and subtrees of children with a parent node, represented as
|
and subtrees of children with a parent node, represented as
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# AVL Tree
|
# AVL Tree
|
||||||
|
|
||||||
In computer science, an AVL tree (named after inventors
|
In computer science, an **AVL tree** (named after inventors
|
||||||
Adelson-Velsky and Landis) is a self-balancing binary search
|
Adelson-Velsky and Landis) is a self-balancing binary search
|
||||||
tree. It was the first such data structure to be invented.
|
tree. It was the first such data structure to be invented.
|
||||||
In an AVL tree, the heights of the two child subtrees of any
|
In an AVL tree, the heights of the two child subtrees of any
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Binary Search Tree
|
# Binary Search Tree
|
||||||
|
|
||||||
In computer science, binary search trees (BST), sometimes called
|
In computer science, **binary search trees** (BST), sometimes called
|
||||||
ordered or sorted binary trees, are a particular type of container:
|
ordered or sorted binary trees, are a particular type of container:
|
||||||
data structures that store "items" (such as numbers, names etc.)
|
data structures that store "items" (such as numbers, names etc.)
|
||||||
in memory. They allow fast lookup, addition and removal of
|
in memory. They allow fast lookup, addition and removal of
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Red–Black Tree
|
# Red–Black Tree
|
||||||
|
|
||||||
A red–black tree is a kind of self-balancing binary search
|
A **red–black tree** is a kind of self-balancing binary search
|
||||||
tree in computer science. Each node of the binary tree has
|
tree in computer science. Each node of the binary tree has
|
||||||
an extra bit, and that bit is often interpreted as the
|
an extra bit, and that bit is often interpreted as the
|
||||||
color (red or black) of the node. These color bits are used
|
color (red or black) of the node. These color bits are used
|
||||||
@ -74,7 +74,7 @@ unlike ordinary binary search trees.
|
|||||||
|
|
||||||
#### Left Right Case (See g, p and x)
|
#### Left Right Case (See g, p and x)
|
||||||
|
|
||||||
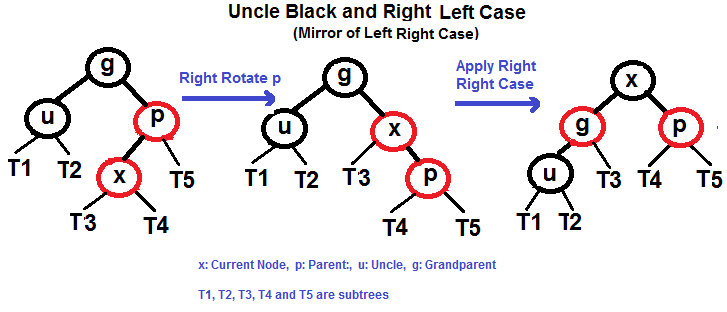
|

|
||||||
|
|
||||||
#### Right Right Case (See g, p and x)
|
#### Right Right Case (See g, p and x)
|
||||||
|
|
||||||
@ -82,7 +82,7 @@ unlike ordinary binary search trees.
|
|||||||
|
|
||||||
#### Right Left Case (See g, p and x)
|
#### Right Left Case (See g, p and x)
|
||||||
|
|
||||||

|
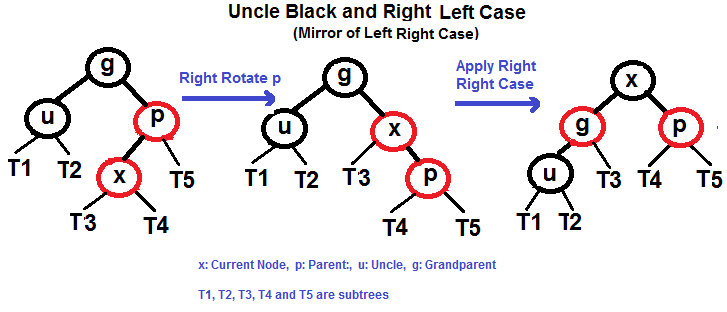
|
||||||
|
|
||||||
## References
|
## References
|
||||||
|
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Segment Tree
|
# Segment Tree
|
||||||
|
|
||||||
In computer science, a segment tree also known as a statistic tree
|
In computer science, a **segment tree** also known as a statistic tree
|
||||||
is a tree data structure used for storing information about intervals,
|
is a tree data structure used for storing information about intervals,
|
||||||
or segments. It allows querying which of the stored segments contain
|
or segments. It allows querying which of the stored segments contain
|
||||||
a given point. It is, in principle, a static structure; that is,
|
a given point. It is, in principle, a static structure; that is,
|
||||||
|
@ -1,6 +1,6 @@
|
|||||||
# Trie
|
# Trie
|
||||||
|
|
||||||
In computer science, a trie, also called digital tree and sometimes
|
In computer science, a **trie**, also called digital tree and sometimes
|
||||||
radix tree or prefix tree (as they can be searched by prefixes),
|
radix tree or prefix tree (as they can be searched by prefixes),
|
||||||
is a kind of search tree—an ordered tree data structure that is
|
is a kind of search tree—an ordered tree data structure that is
|
||||||
used to store a dynamic set or associative array where the keys
|
used to store a dynamic set or associative array where the keys
|
||||||
|
Loading…
Reference in New Issue
Block a user