mirror of
https://github.moeyy.xyz/https://github.com/trekhleb/javascript-algorithms.git
synced 2024-12-24 21:46:13 +08:00
docs: update correct Big-O chart (#62)
This commit is contained in:
parent
9de6bc7de3
commit
bc17e4ea2c
32
README.md
32
README.md
@ -3,22 +3,22 @@
|
||||
[](https://travis-ci.org/trekhleb/javascript-algorithms)
|
||||
[](https://codecov.io/gh/trekhleb/javascript-algorithms)
|
||||
|
||||
This repository contains JavaScript based examples of many
|
||||
This repository contains JavaScript based examples of many
|
||||
popular algorithms and data structures.
|
||||
|
||||
Each algorithm and data structure has its own separate README
|
||||
with related explanations and links for further reading (including ones
|
||||
to YouTube videos).
|
||||
|
||||
_Read this in other languages:_
|
||||
_Read this in other languages:_
|
||||
[简体中文](https://github.com/trekhleb/javascript-algorithms/blob/master/README.zh-CN.md),
|
||||
[繁體中文](https://github.com/trekhleb/javascript-algorithms/blob/master/README.zh-TW.md)
|
||||
|
||||
## Data Structures
|
||||
|
||||
A data structure is a particular way of organizing and storing data in a computer so that it can
|
||||
be accessed and modified efficiently. More precisely, a data structure is a collection of data
|
||||
values, the relationships among them, and the functions or operations that can be applied to
|
||||
be accessed and modified efficiently. More precisely, a data structure is a collection of data
|
||||
values, the relationships among them, and the functions or operations that can be applied to
|
||||
the data.
|
||||
|
||||
* [Linked List](https://github.com/trekhleb/javascript-algorithms/tree/master/src/data-structures/linked-list)
|
||||
@ -59,7 +59,7 @@ a set of rules that precisely define a sequence of operations.
|
||||
* [Permutations](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/permutations) (with and without repetitions)
|
||||
* [Combinations](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/combinations) (with and without repetitions)
|
||||
* [Fisher–Yates Shuffle](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/fisher-yates) - random permutation of a finite sequence
|
||||
* [Longest Common Subsequence](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-common-subsequnce) (LCS)
|
||||
* [Longest Common Subsequence](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-common-subsequnce) (LCS)
|
||||
* [Longest Increasing subsequence](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-increasing-subsequence)
|
||||
* [Shortest Common Supersequence](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/shortest-common-supersequence) (SCS)
|
||||
* [Knapsack Problem](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/knapsack-problem) - "0/1" and "Unbound" ones
|
||||
@ -105,11 +105,11 @@ a set of rules that precisely define a sequence of operations.
|
||||
* [Tower of Hanoi](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/hanoi-tower)
|
||||
* [N-Queens Problem](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/n-queens)
|
||||
* [Knight's Tour](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/knight-tour)
|
||||
|
||||
|
||||
### Algorithms by Paradigm
|
||||
|
||||
An algorithmic paradigm is a generic method or approach which underlies the design of a class
|
||||
of algorithms. It is an abstraction higher than the notion of an algorithm, just as an
|
||||
An algorithmic paradigm is a generic method or approach which underlies the design of a class
|
||||
of algorithms. It is an abstraction higher than the notion of an algorithm, just as an
|
||||
algorithm is an abstraction higher than a computer program.
|
||||
|
||||
* **Brute Force** - look at all the possibilities and selects the best solution
|
||||
@ -142,7 +142,7 @@ algorithm is an abstraction higher than a computer program.
|
||||
* [Maximum Subarray](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/maximum-subarray)
|
||||
* [Bellman-Ford Algorithm](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/graph/bellman-ford) - finding shortest path to all graph vertices
|
||||
* **Backtracking** - similarly to brute force, try to generate all possible solutions, but each time you generate next solution you test
|
||||
if it satisfies all conditions, and only then continue generating subsequent solutions. Otherwise, backtrack, and go on a
|
||||
if it satisfies all conditions, and only then continue generating subsequent solutions. Otherwise, backtrack, and go on a
|
||||
different path of finding a solution. Normally the DFS traversal of state-space is being used.
|
||||
* [Hamiltonian Cycle](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/graph/hamiltonian-cycle) - Visit every vertex exactly once
|
||||
* [N-Queens Problem](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/n-queens)
|
||||
@ -188,13 +188,13 @@ npm test -- -t 'playground'
|
||||
[▶ Data Structures and Algorithms on YouTube](https://www.youtube.com/playlist?list=PLLXdhg_r2hKA7DPDsunoDZ-Z769jWn4R8)
|
||||
|
||||
### Big O Notation
|
||||
|
||||
|
||||
Order of growth of algorithms specified in Big O notation.
|
||||
|
||||
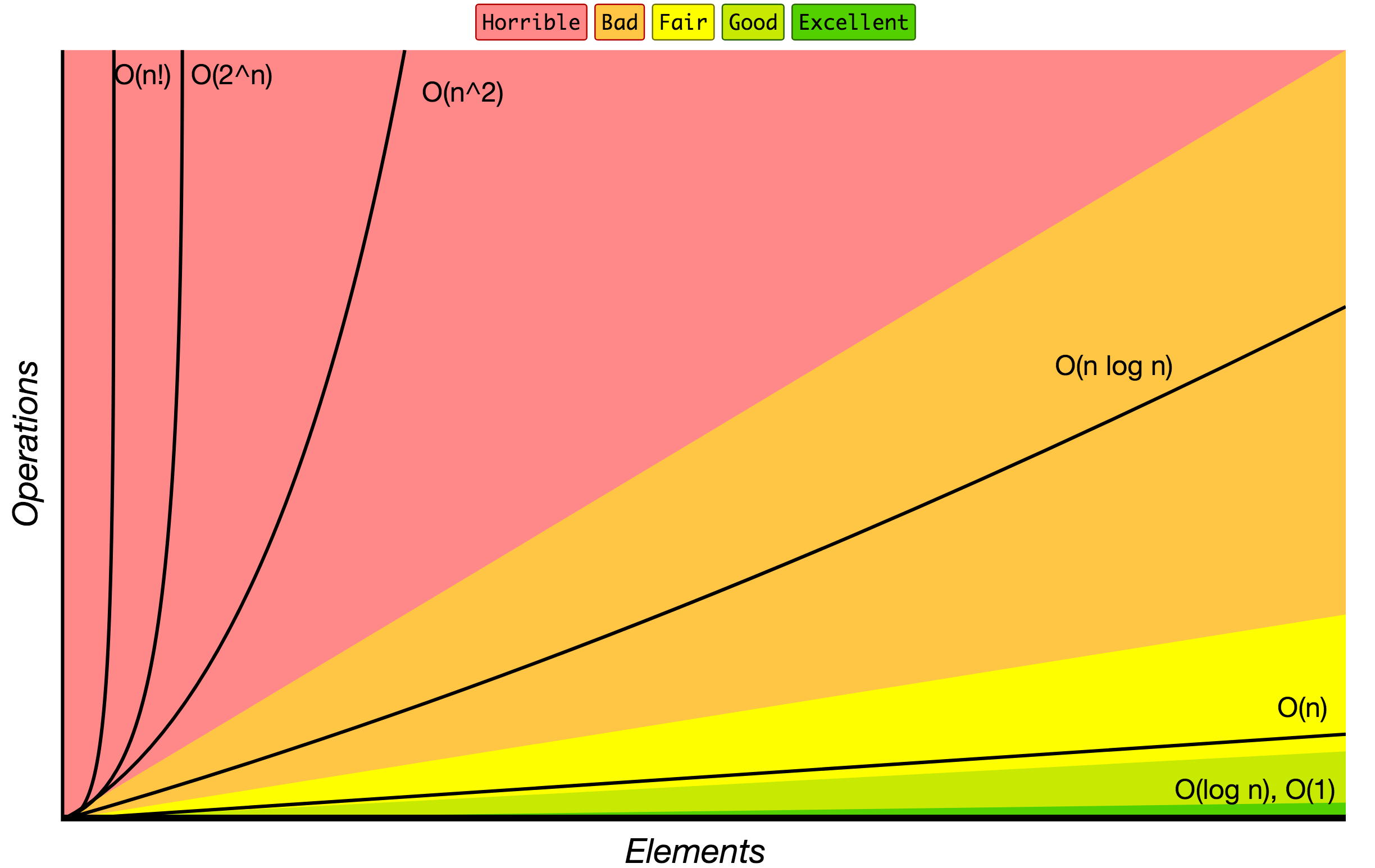
|
||||
|
||||

|
||||
|
||||
Source: [Big O Cheat Sheet](http://bigocheatsheet.com/).
|
||||
|
||||
|
||||
Below is the list of some of the most used Big O notations and their performance comparisons against different sizes of the input data.
|
||||
|
||||
| Big O Notation | Computations for 10 elements | Computations for 100 elements | Computations for 1000 elements |
|
||||
@ -208,12 +208,12 @@ Below is the list of some of the most used Big O notations and their performance
|
||||
| **O(N!)** | 3628800 | 9.3e+157 | 4.02e+2567 |
|
||||
|
||||
### Data Structure Operations Complexity
|
||||
|
||||
|
||||
| Data Structure | Access | Search | Insertion | Deletion | Comments |
|
||||
| ----------------------- | :-------: | :-------: | :-------: | :-------: | :-------- |
|
||||
| ----------------------- | :-------: | :-------: | :-------: | :-------: | :-------- |
|
||||
| **Array** | 1 | n | n | n | |
|
||||
| **Stack** | n | n | 1 | 1 | |
|
||||
| **Queue** | n | n | 1 | 1 | |
|
||||
| **Queue** | n | n | 1 | 1 | |
|
||||
| **Linked List** | n | n | 1 | 1 | |
|
||||
| **Hash Table** | - | n | n | n | In case of perfect hash function costs would be O(1) |
|
||||
| **Binary Search Tree** | n | n | n | n | In case of balanced tree costs would be O(log(n)) |
|
||||
|
@ -170,7 +170,7 @@ npm test -- -t 'playground'
|
||||
|
||||
大O符号中指定的算法的增长顺序。
|
||||
|
||||
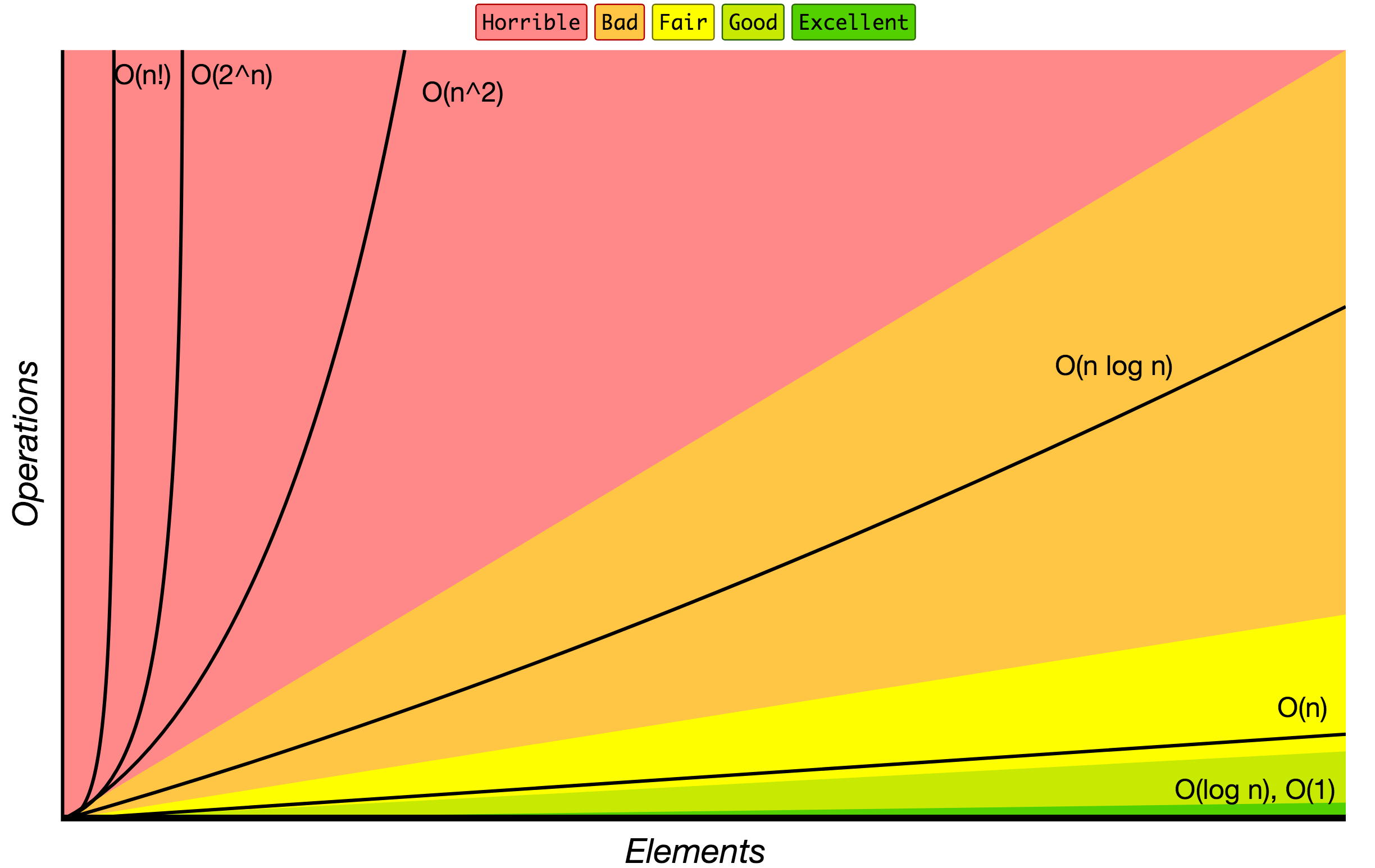
|
||||

|
||||
|
||||
源: [Big O Cheat Sheet](http://bigocheatsheet.com/).
|
||||
|
||||
|
@ -47,7 +47,7 @@ _Read this in other languages:_
|
||||
* [排列](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/permutations) (有/無重複)
|
||||
* [组合](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/combinations) (有/無重複)
|
||||
* [洗牌算法](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/fisher-yates) - 隨機置換一有限序列
|
||||
* [最長共同子序列](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-common-subsequnce) (LCS)
|
||||
* [最長共同子序列](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-common-subsequnce) (LCS)
|
||||
* [最長遞增子序列](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/longest-increasing-subsequence)
|
||||
* [Shortest Common Supersequence](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/shortest-common-supersequence) (SCS)
|
||||
* [背包問題](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/sets/knapsack-problem) - "0/1" and "Unbound" ones
|
||||
@ -90,7 +90,7 @@ _Read this in other languages:_
|
||||
* [河內塔](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/hanoi-tower)
|
||||
* [N-皇后問題](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/n-queens)
|
||||
* [騎士走棋盤](https://github.com/trekhleb/javascript-algorithms/tree/master/src/algorithms/uncategorized/knight-tour)
|
||||
|
||||
|
||||
### 演算法範型
|
||||
|
||||
演算法的範型是一個泛用方法或設計一類底層演算法的方式。它是一個比演算法的概念更高階的抽象化,就像是演算法是比電腦程式更高階的抽象化。
|
||||
@ -166,11 +166,11 @@ npm test -- -t 'playground'
|
||||
### 大 O 標記
|
||||
|
||||
特別用大 O 標記演算法增長度的排序。
|
||||
|
||||
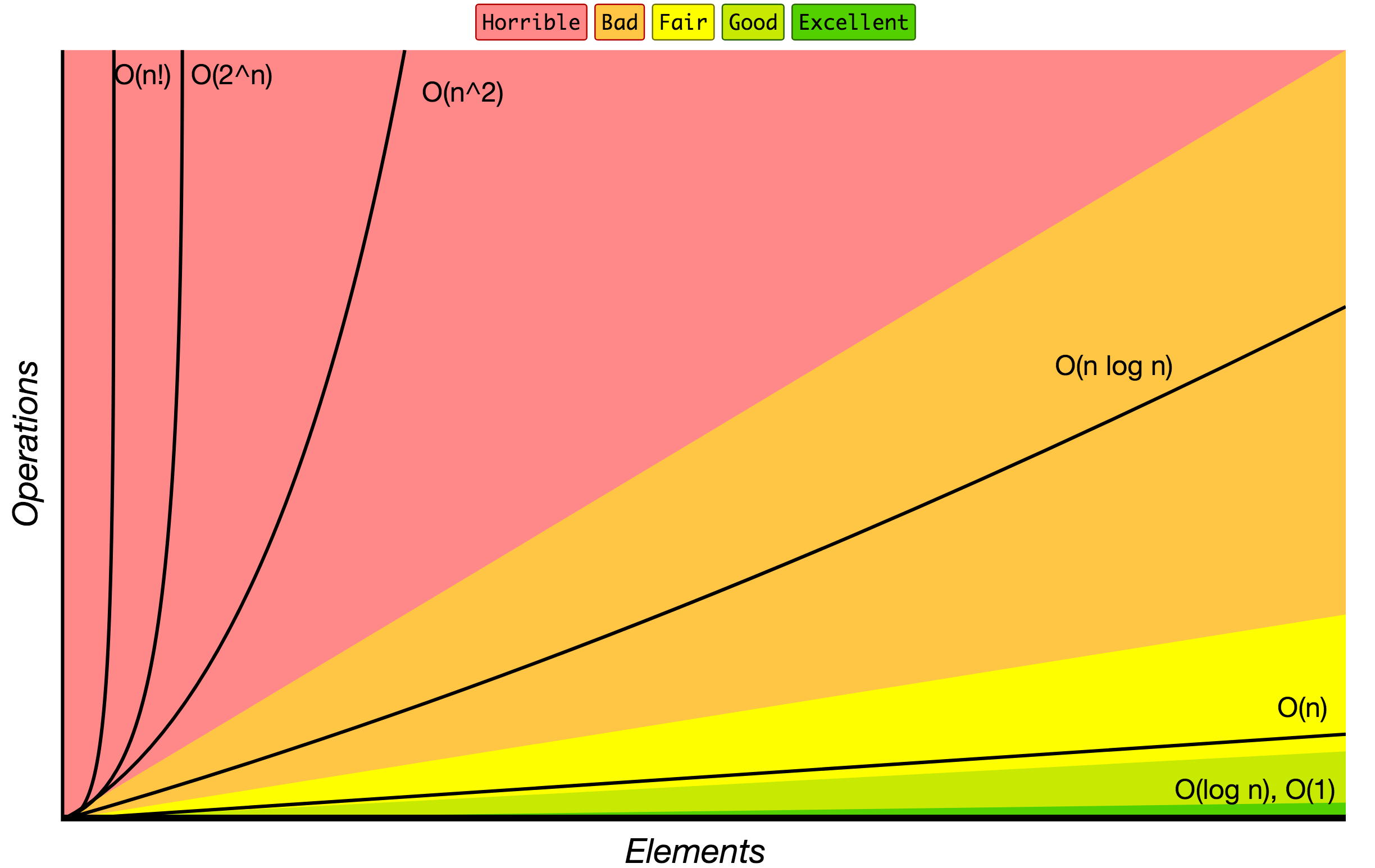
|
||||
|
||||

|
||||
|
||||
資料來源: [Big O Cheat Sheet](http://bigocheatsheet.com/).
|
||||
|
||||
|
||||
下列列出幾個常用的 Big O 標記以及其不同大小資料量輸入後的運算效能比較。
|
||||
|
||||
| Big O 標記 | 10個資料量需花費的時間 | 100個資料量需花費的時間 | 1000個資料量需花費的時間 |
|
||||
@ -184,12 +184,12 @@ npm test -- -t 'playground'
|
||||
| **O(N!)** | 3628800 | 9.3e+157 | 4.02e+2567 |
|
||||
|
||||
### 資料結構運作複雜度
|
||||
|
||||
|
||||
| 資料結構 | 存取 | 搜尋 | 插入 | 刪除 |
|
||||
| ----------------------- | :-------: | :-------: | :-------: | :-------: |
|
||||
| ----------------------- | :-------: | :-------: | :-------: | :-------: |
|
||||
| **陣列** | 1 | n | n | n |
|
||||
| **堆疊** | n | n | 1 | 1 |
|
||||
| **貯列** | n | n | 1 | 1 |
|
||||
| **貯列** | n | n | 1 | 1 |
|
||||
| **鏈結串列** | n | n | 1 | 1 |
|
||||
| **雜湊表** | - | n | n | n |
|
||||
| **二元搜尋樹** | n | n | n | n |
|
||||
|
Binary file not shown.
Before Width: | Height: | Size: 89 KiB After Width: | Height: | Size: 60 KiB |
Loading…
Reference in New Issue
Block a user