mirror of
https://github.moeyy.xyz/https://github.com/trekhleb/javascript-algorithms.git
synced 2024-09-20 07:43:04 +08:00
Compare commits
4 Commits
dd25cba727
...
f6386ffe98
Author | SHA1 | Date | |
---|---|---|---|
![]() |
f6386ffe98 | ||
![]() |
1fc9f6447e | ||
![]() |
80e4d05ae1 | ||
![]() |
f1c261695a |
39
src/algorithms/sorting/Bucket-sort/BucketSort.js
Normal file
39
src/algorithms/sorting/Bucket-sort/BucketSort.js
Normal file
@ -0,0 +1,39 @@
|
||||
import Sort from '../Sort';
|
||||
|
||||
export default class BucketSort extends Sort {
|
||||
sort(originalArray) {
|
||||
let array = [];
|
||||
let bucketSize = originalArray.length;
|
||||
|
||||
let minValue = originalArray[0];
|
||||
let maxValue = originalArray[0];
|
||||
originalArray.forEach(function (currentVal) { //get Min and Max number in array
|
||||
if (currentVal < minValue) {
|
||||
minValue = currentVal;
|
||||
} else if (currentVal > maxValue) {
|
||||
maxValue = currentVal;
|
||||
}
|
||||
})
|
||||
|
||||
let buckets = new Array(bucketSize);
|
||||
|
||||
for (var i = 0; i < buckets.length; i++) { //make 2d array for storing numbers in different buckets
|
||||
buckets[i] = new Array(bucketSize);
|
||||
}
|
||||
|
||||
originalArray.forEach(element => { //for each item in array sort them into their respected bucket
|
||||
let index = Math.floor(element - minValue); // get index by getting the number - min value in array.
|
||||
buckets[index].push(element); //pushes element into bucket of index we found
|
||||
});
|
||||
|
||||
buckets.forEach(element => { // Concat all buckets into one array
|
||||
element.forEach(number => {
|
||||
if (typeof number !== 'undefined') {
|
||||
array.push(number);
|
||||
}
|
||||
});
|
||||
});
|
||||
|
||||
return array
|
||||
}
|
||||
}
|
42
src/algorithms/sorting/Bucket-sort/README.md
Normal file
42
src/algorithms/sorting/Bucket-sort/README.md
Normal file
@ -0,0 +1,42 @@
|
||||
# Bucket Sort
|
||||
|
||||
**Bucket sort** is a sorting algorithm that works by distributing
|
||||
elements of an array into a number of different buckets.Each bucket
|
||||
representing a number range, then the buckets are sorted individually,
|
||||
either using a different sorting algorithm, or by recursively applying
|
||||
the bucket sorting algorithm.
|
||||
|
||||
The average time complexity for Bucket Sort is O(n + k) (k = number of buckets). The worst time
|
||||
complexity is O(n²).
|
||||
|
||||
## Algorithm
|
||||
|
||||
**Step I**
|
||||
|
||||
Our first real step is to create our buckets, we make an array thats the size of our original array, and then that array into a 2D array that will represent our buckets. This array will hold all of our elements for when we sort them.
|
||||
|
||||
<!-- 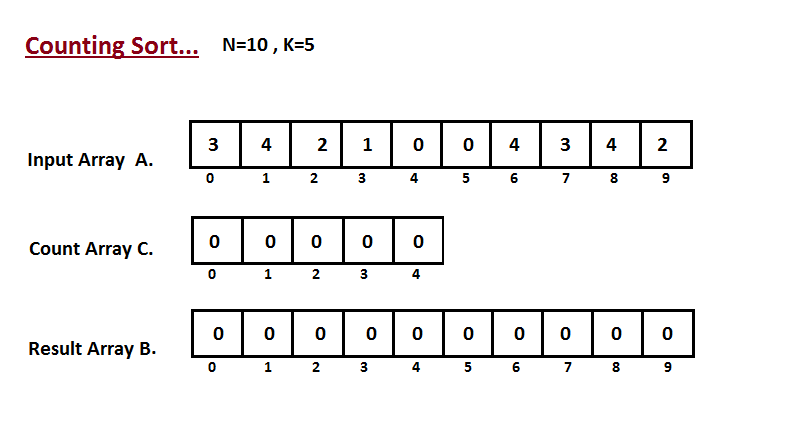 -->
|
||||
|
||||
**Step II**
|
||||
|
||||
Next step we do the sorting, we find the index of the bucket we want to add our value to by doing a small equation. We take the element we want to sort and subtract the smallest value from our original array, and thats our index, so we just push that to our buckets array.
|
||||
|
||||
<!-- 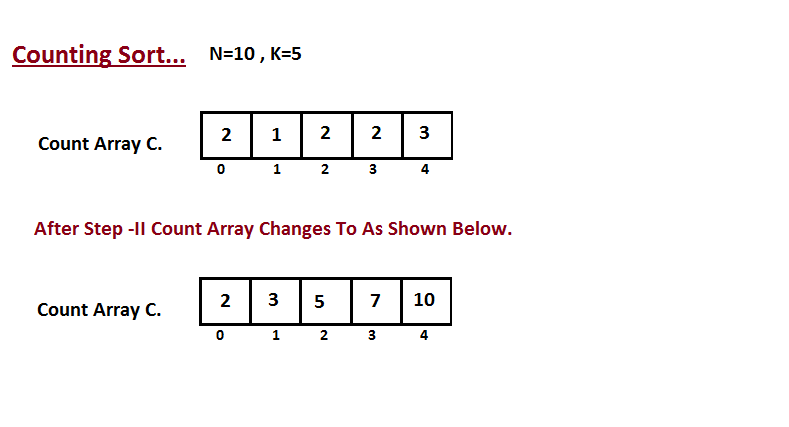 -->
|
||||
|
||||
**Step III**
|
||||
|
||||
Our last and final step is to compine all of our buckets to one array so we can return the sorted array.
|
||||
|
||||
<!-- 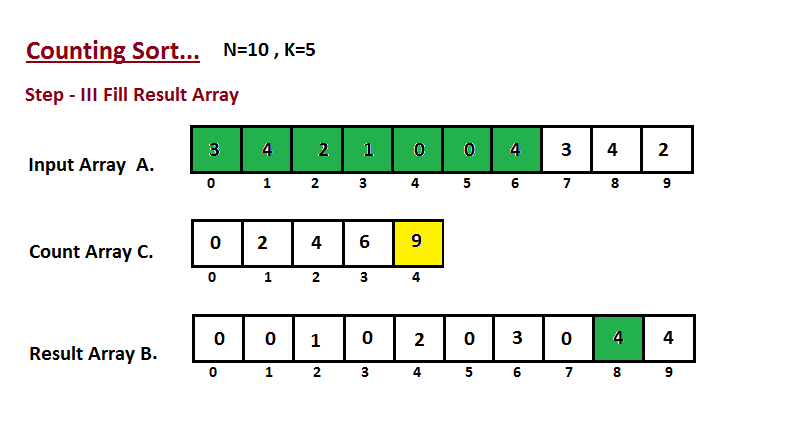 -->
|
||||
|
||||
## Complexity
|
||||
|
||||
| Name | Best | Average | Worst | Memory | Stable | Comments |
|
||||
| --------------------- | :-------------: | :-----------------: | :-----------------: | :-------: | :-------: | :-------- |
|
||||
| **Counting sort** | n | n + k | n^2 | n + k | Yes | k - number of buckets |
|
||||
|
||||
## References
|
||||
|
||||
- [Wikipedia](https://en.wikipedia.org/wiki/Bucket_sort)
|
||||
- [YouTube](https://www.youtube.com/watch?v=VuXbEb5ywrU)
|
||||
- [GeeksforGeeks](https://www.geeksforgeeks.org/bucket-sort-2/)
|
@ -0,0 +1,64 @@
|
||||
import BucketSort from '../BucketSort';
|
||||
import {
|
||||
equalArr,
|
||||
notSortedArr,
|
||||
reverseArr,
|
||||
sortedArr,
|
||||
SortTester,
|
||||
} from '../../SortTester';
|
||||
|
||||
// Complexity constants.
|
||||
// const SORTED_ARRAY_VISITING_COUNT = 20;
|
||||
// const NOT_SORTED_ARRAY_VISITING_COUNT = 189;
|
||||
// const REVERSE_SORTED_ARRAY_VISITING_COUNT = 209;
|
||||
// const EQUAL_ARRAY_VISITING_COUNT = 20;
|
||||
|
||||
describe('BucketSort', () => {
|
||||
it('should sort array', () => {
|
||||
SortTester.testSort(BucketSort);
|
||||
});
|
||||
|
||||
// it('should sort array with custom comparator', () => {
|
||||
// SortTester.testSortWithCustomComparator(BucketSort);
|
||||
// });
|
||||
|
||||
// it('should do stable sorting', () => {
|
||||
// SortTester.testSortStability(BucketSort);
|
||||
// });
|
||||
|
||||
// it('should sort negative numbers', () => {
|
||||
// SortTester.testNegativeNumbersSort(BucketSort);
|
||||
// });
|
||||
|
||||
// it('should visit EQUAL array element specified number of times', () => {
|
||||
// SortTester.testAlgorithmTimeComplexity(
|
||||
// BucketSort,
|
||||
// equalArr,
|
||||
// EQUAL_ARRAY_VISITING_COUNT,
|
||||
// );
|
||||
// });
|
||||
|
||||
// it('should visit SORTED array element specified number of times', () => {
|
||||
// SortTester.testAlgorithmTimeComplexity(
|
||||
// BucketSort,
|
||||
// sortedArr,
|
||||
// SORTED_ARRAY_VISITING_COUNT,
|
||||
// );
|
||||
// });
|
||||
|
||||
// it('should visit NOT SORTED array element specified number of times', () => {
|
||||
// SortTester.testAlgorithmTimeComplexity(
|
||||
// BucketSort,
|
||||
// notSortedArr,
|
||||
// NOT_SORTED_ARRAY_VISITING_COUNT,
|
||||
// );
|
||||
// });
|
||||
|
||||
// it('should visit REVERSE SORTED array element specified number of times', () => {
|
||||
// SortTester.testAlgorithmTimeComplexity(
|
||||
// BucketSort,
|
||||
// reverseArr,
|
||||
// REVERSE_SORTED_ARRAY_VISITING_COUNT,
|
||||
// );
|
||||
// });
|
||||
});
|
Loading…
Reference in New Issue
Block a user